DRY vs WET Code: A Comparative Study with Python and Go
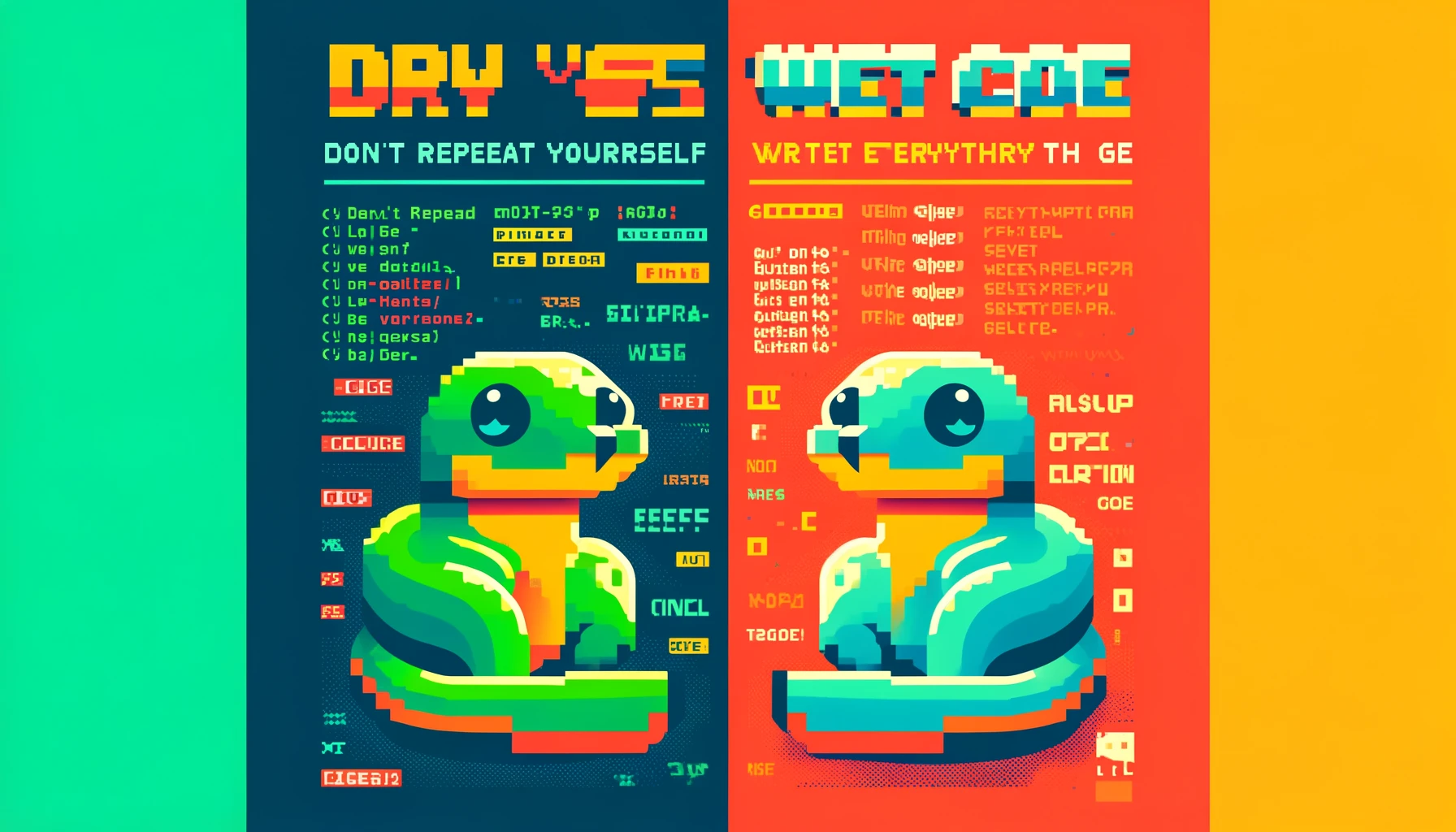
DRY vs WET Code: A Comparative Study with Python and Go
Introduction
The DRY principle, which stands for “Don’t Repeat Yourself,” is a fundamental concept in software development aimed at reducing repetition of software patterns, replacing it with abstractions or using data normalization to avoid redundancy. This article provides an in-depth look at the DRY principle, its implementation in Python and Go, and its application in software design using UML diagrams.
Understanding DRY
The DRY principle was formulated by Andy Hunt and Dave Thomas in their book “The Pragmatic Programmer.” It states:
“Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.”
This means that in a well-designed system, each piece of functionality should be implemented in just one place. The goal is to avoid duplicating code and data, which can lead to maintenance nightmares, poor factoring, and logical contradictions.
Benefits of DRY
- Improved Maintainability: Changes need to be made in only one place.
- Reduced Complexity: Less code generally means less complexity.
- Enhanced Readability: Code is more concise and often clearer.
- Easier Testing: With functionality centralized, testing becomes more straightforward.
- Better Scalability: DRY code is often more modular and easier to scale.
DRY vs WET Code
WET stands for “Write Everything Twice” or “We Enjoy Typing.” It’s the antithesis of DRY. Let’s compare:
|
|
In the WET example, we have two nearly identical functions. In the DRY version, we have a single function that can be reused.
Implementing DRY in Python
Let’s look at a more complex example in Python, this time using a banking system:
|
|
In this DRY version, we create a base BankAccount
class with common functionality, and then create SavingsAccount
and CheckingAccount
as subclasses. This eliminates code duplication and allows for easy extension with account-specific features.
Implementing DRY in Go
Now let’s see how we can apply DRY in Go using a different example - a simple task management system:
|
|
In this DRY version, we define a Task
interface and a BaseTask
struct with common functionality. The WorkTask
and PersonalTask
types embed BaseTask
and implement the Task
interface, allowing for code reuse while maintaining type-specific behavior.
DRY in Software Design: UML Examples
Let’s update our UML diagram to reflect the new banking system example:
┌───────────────┐
│ BankAccount │
├───────────────┤
│-accountNumber │
│-balance │
├───────────────┤
│+deposit() │
│+withdraw() │
└───────────────┘
▲
│
├───────────────┐
│ │
┌─────────────────┐ ┌───────────────┐
│SavingsAccount │ │CheckingAccount│
├─────────────────┤ ├───────────────┤
│-interestRate │ │-overdraftLimit│
├─────────────────┤ ├───────────────┤
│+applyInterest() │ │+withdraw() │
└─────────────────┘ └───────────────┘
This UML diagram shows how the SavingsAccount
and CheckingAccount
classes inherit from the BankAccount
base class, demonstrating the DRY principle in the class hierarchy.
Common Pitfalls and Misconceptions
- Over-DRYing: Sometimes, trying to eliminate all repetition can lead to overly complex abstractions.
- Premature Abstraction: Applying DRY before fully understanding the problem domain can result in incorrect abstractions.
- Ignoring Semantic Differences: Two pieces of code may look similar but serve different purposes. Blindly combining them might be a mistake.
Best Practices for Applying DRY
- Identify Common Patterns: Look for recurring code or logic in your system.
- Create Abstractions Thoughtfully: Ensure your abstractions truly represent the problem domain.
- Use Inheritance and Composition: Leverage OOP principles to avoid repetition.
- Utilize Functions and Methods: Extract common logic into reusable functions.
- Apply DRY to Data: Use normalization techniques in databases to avoid data redundancy.
- Refactor Regularly: Continuously look for opportunities to apply DRY as your codebase evolves.
Conclusion
The DRY principle is a powerful tool for creating maintainable, scalable, and efficient code. By avoiding repetition and creating meaningful abstractions, developers can significantly improve the quality of their software. However, it’s crucial to apply DRY thoughtfully, always considering the specific context and requirements of your project. Remember, the goal is not to eliminate all repetition, but to ensure that every piece of knowledge has a single, authoritative representation in your system.